Table Of Content

I hope to receive contributions from everyone so that the programming community can continue to develop, and especially so that future articles can be better. First, we create a BankAccount, which is an abstract class that will contain information commonly used for specific Accounts. Lets you define a subscription mechanism to notify multiple objects about any events that happen to the object they're observing. A proxy controls access to the original object, allowing you to perform something either before or after the request gets through to the original object. Model-View-Controller (MVC) Pattern is one of the oldest architectural patterns for creating web applications. The facade pattern is used to help client applications easily interact with the system.
Behavioral Design Patterns in Java
If object creation code is spread in the whole application, and if you need to change the process of object creation then you need to go in each and every place to make necessary changes. After finishing this article, while writing your application, consider using the Java factory pattern. As you can see, the creation of objects in the method create(AccountType type) depends on the constructor of the sub-classes. Therefore, if the constructor has many parameters, the factory method can become complex. The adapter design pattern is one of the structural design patterns and is used so that two unrelated interfaces can work together.
Step 3: Define the Factory Interface
It will help us in making any kind of changes in car making process without even touching the composing classes i.e. classes using CarFactory. As long as all product classes implement a common interface, you can pass their objects to the client code without breaking it. In this article, I am going to discuss the Factory Design Pattern in Java with Real-Time Examples.
How to Implement the Factory Design Pattern ❓
It allows you to create objects without specifying their exact types, delegating the responsibility to subclasses or specialized factory classes. The Factory Design Pattern is a design pattern that provides a single interface for creating objects, with the implementation of the object creation process being handled by a factory class. This factory class is responsible for instantiating objects based on a set of conditions or parameters that are passed to it by the client code. The Factory Design Pattern is a creational design pattern that provides a simple and flexible way to create objects, decoupling the process of object creation from the client code. This pattern can be used to create objects of a single type, or objects of different types based on a set of conditions. In this article, we will explore the Factory Design Pattern in detail and look at its various use cases and implementation techniques.
This pattern promotes modularity, extensibility, and code reusability, making it a valuable tool in software development. The Factory Method pattern allows subclasses to choose the type of objects to create without revealing the creation process to the client. It provides an interface for creating objects in a way that is convenient and flexible for software development. It states the best way to create an object without telling the exact class of object that will be created. It is used when we have a super-class with multiple sub-classes and based on input, we want to return one of the sub-class.
Step 1
Pankaj, You have a wide reach and your article make a huge impact on developers. I appreciate your work and dedication that you put to bring this in front of us all. Having said that I want to invite you to partner me in clearing the space and providing the correct Design patterns as they are and not as they occur to you, me or any other author. I want to point out that the example you have put is neither of that.
To instantiate car objects, a GetFuelFactory class can be implemented. This class will have methods for creating different types of fuel based on the client’s request. The iterator pattern is one of the behavioral patterns and is used to provide a standard way to traverse through a group of objects. The iterator pattern is widely used in Java Collection Framework where the iterator interface provides methods for traversing through a Collection.
This pattern decouples object creation from the client code, promoting flexibility, maintainability, and scalability. The Factory design pattern is intended to define an interface for creating an object, but allows subclasses to alter the type of objects that will be created. This pattern is particularly useful when the creation process involves complexity. Factory Method is a creational design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.
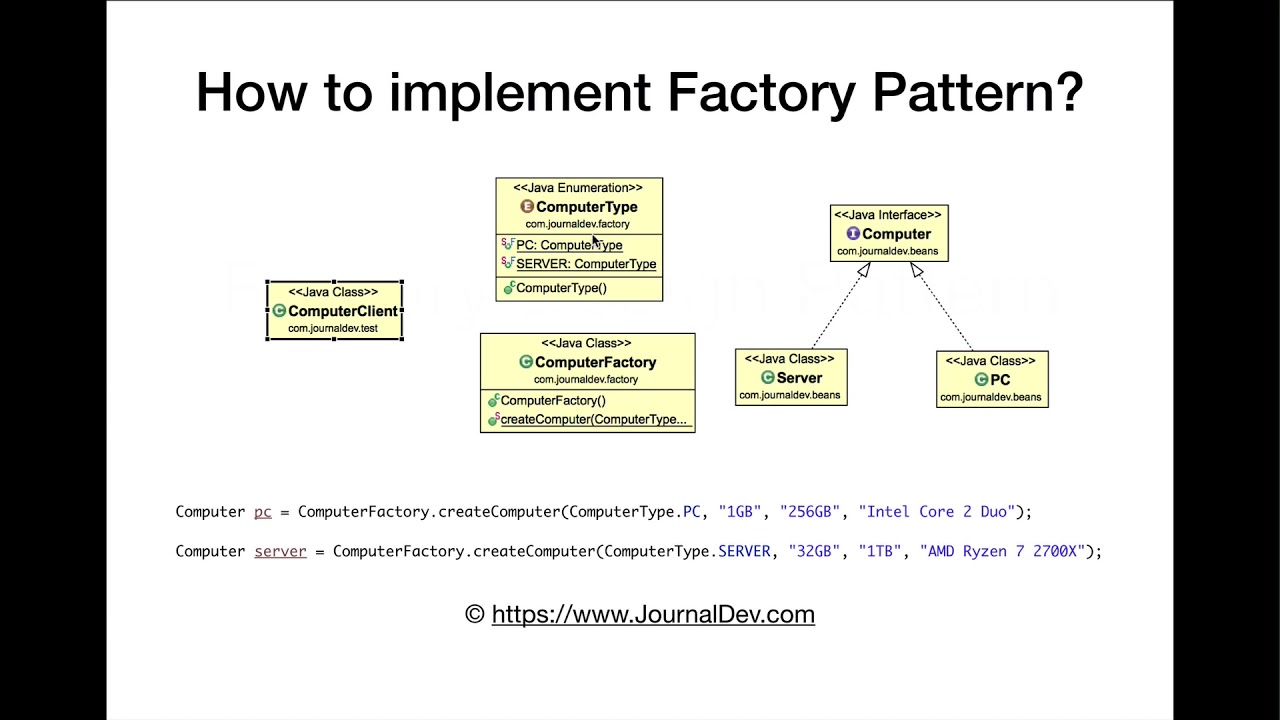
Later, based on the order received, the appropriate product is delivered by the factory. If you are ordering a car, then based on your requirements or specifications, the factory will create the appropriate car and then deliver that car to you. All those tutorials for beginners java developers (yt, udemy,…) are created usually in which design pattern? On all tutorials which I use, free and bought I never heard any of those teacher that they are mentioned any design pattern. I know they exist but how I see at the interview they ask about them. The Data Access Object (DAO) design pattern is used to decouple the data persistence logic to a separate layer.
Source code and design conformance, design pattern detection from source code by classification approach - ScienceDirect.com
Source code and design conformance, design pattern detection from source code by classification approach.
Posted: Fri, 11 Oct 2019 05:39:09 GMT [source]
To ensure that these steps are centralized and not exposed to composing classes, factory pattern should be used. We can see many realtime examples of factory pattern in JDK itself e.g. It also prevents us in making changes to car making process because the code is not centralized, and making changes in all composing classes seems not feasible. For example, in the future, there might be new types of accounts such as Credit, Debit, etc. At that time, we only need to create additional subclasses that extend BankAccount and add them to the factory method.
Introduction to RxJava: Observable Pattern - hackernoon.com
Introduction to RxJava: Observable Pattern.
Posted: Thu, 26 Mar 2020 07:00:00 GMT [source]
With the Factory Pattern, the object creation logic is hidden from the client. Instead of knowing the exact object class and instantiating it through a constructor, the responsibility of creating an object is moved away from the client. Factory, as the name suggests, is a place to create some different products which are somehow similar in features yet divided into categories. Here is a simple test client program that uses above factory design pattern implementation. Super class in factory design pattern can be an interface, abstract class or a normal java class. For our factory design pattern example, we have abstract super class with overridden toString() method for testing purpose.
In this article, we will see what is the intent of the Factory design pattern, what problems it solves, and its applicability. Design patterns are a collection of programming methodologies used in day-to-day programming. They represent solutions to some commonly occurring problems in the programming industry, which have intuitive solutions.
Turns a request into a stand-alone object that contains all information about the request. This transformation lets you pass requests as a method arguments, delay or queue a request's execution, and support undoable operations. Upon receiving a request, each handler decides either to process the request or to pass it to the next handler in the chain. Lets you attach new behaviors to objects by placing these objects inside special wrapper objects that contain the behaviors. Lets you compose objects into tree structures and then work with these structures as if they were individual objects. Lets you ensure that a class has only one instance, while providing a global access point to this instance.
We have an interface Coin and two implementations GoldCoin and CopperCoin. Javatpoint provides tutorials with examples, code snippets, and practical insights, making it suitable for both beginners and experienced developers. If you can’t figure out the difference between various factory patterns and concepts, then read our Factory Comparison. This goes on as you add more related variations of a logical collection - all spaceships for instance.
Factory methods pervade toolkits and frameworks.The preceding document example is a typical use in MacApp and ET++. Memento pattern is implemented with two Objects – originator and caretaker. The originator is the Object whose state needs to be saved and restored, and it uses an inner class to save the state of Object. The inner class is called “Memento”, and it’s private so that it can’t be accessed from other objects. One of the best examples of this pattern is the Collections.sort() method that takes the Comparator parameter.
The client can then create objects through a common interface which simplifies the process. Therefore, you need to have a regular method capable of creating new objects as well as reusing existing ones. Probably the most obvious and convenient place where this code could be placed is the constructor of the class whose objects we’re trying to reuse.
No comments:
Post a Comment